Understanding Laravel Folder Structure
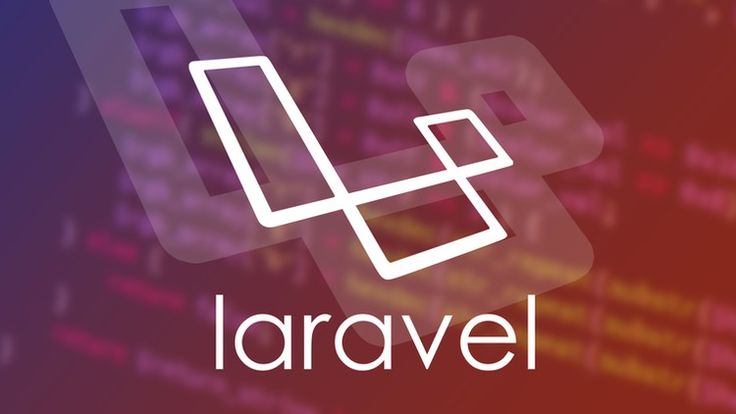
Laravel is one of the most popular PHP frameworks for building modern, robust web applications. One of the key features that makes Laravel so powerful and developer-friendly is its well-organized folder structure. Therefore, understanding this folder structure will help you navigate your project more efficiently, write clean code, and work effectively with your team.
In this blog, we will dive into Laravel’s folder structure and explain the purpose of each folder. As a result, you will understand where specific files should go and how Laravel keeps things neatly organized.
Laravel Folder Structure Overview
When you create a new Laravel project, the default folder structure is already set up for you. Here’s an overview of the default Laravel folder structure:
laravel-project/
app/
bootstrap/
config/
database/
public/
resources/
routes/
storage/
tests/
vendor/
Each of these folders has a specific role in the framework. Consequently, knowing their purpose will help you maintain a well-structured application. Let’s break it down:
Before diving into the folder structure, ensure you have set up Laravel correctly. Follow Laravel installation guide for step-by-step instructions.
1. app/
– The Core Application Folder
The app/
folder is the heart of your Laravel application. It contains the core logic and functionality of your app. This is where you will spend most of your time writing business logic, interacting with databases, and managing how the application responds to requests.
Inside the app/
folder, you’ll find several subfolders:
Http/
: This folder contains all your HTTP-related logic.Controllers/
: Stores the controllers that handle incoming requests.Middleware/
: Contains middleware classes that filter HTTP requests.Requests/
: Holds custom request classes for validating incoming requests.
Models/
: This is where your Eloquent models live. Since Eloquent is Laravel’s ORM (Object-Relational Mapper), it allows you to interact with your database tables as if they were PHP objects.Providers/
: Contains service providers, which are responsible for binding services into Laravel’s service container.
In short, the app/
folder is where all the application’s core logic and features reside.
2. bootstrap/
– Bootstrap Files for the Application
The bootstrap/
folder contains files necessary for bootstrapping the Laravel framework and initializing your application. This folder is mainly used for setup tasks like defining the environment settings and configuring autoloading.
app.php
: This file is the starting point for Laravel’s request lifecycle. It initializes the Laravel framework and loads your application.cache/
: Stores cached files to improve performance. Generally, you don’t need to interact with this folder directly.
3. config/
– Configuration Files
The config/
folder contains all of your application’s configuration files. Here, you can adjust settings for things like the database, mail, caching, and more. Each file in this folder corresponds to a different aspect of your application.
For example:
app.php
: Contains global configuration settings like the application name, timezone, and environment.database.php
: Configures your database connection settings (MySQL, SQLite, etc.).mail.php
: Configures the mail service.
Thus, you will likely find yourself modifying some of these files during your development process.
4. database/
– Database Migrations, Factories, and Seeders
The database/
folder holds all files related to the database. Specifically, it includes:
migrations/
: Migrations act as version control for your database schema. They help create, modify, and update your database tables.factories/
: Laravel factories allow you to quickly generate fake data for testing purposes. For instance, you can create fake blog posts, users, or comments.seeders/
: Seeders populate the database with default data. For example, you might create a seeder to insert sample records like default categories or blog posts.
5. public/
– The Web Root Folder
The public/
folder is the only folder accessible to the web. It contains front-end assets and acts as the entry point for all web traffic.
index.php
: The front controller that handles all incoming HTTP requests.assets/
: Stores your CSS, JavaScript, and image files.robots.txt
: Guides search engine crawlers.
Since the public/
folder is the only accessible directory, keeping internal folders safe from public access is crucial.
6. resources/
– Views, Language Files, and Assets
The resources/
folder contains all your raw, uncompiled files. Inside this folder, you’ll find:
views/
: Stores Blade templates, Laravel’s templating engine.lang/
: Holds localization files for multi-language support.assets/
: Contains raw CSS, JavaScript, and images. Laravel uses tools like Laravel Mix to compile these files for production.
7. routes/
– Defining Routes
The routes/
folder defines all the application’s routes. This is essential because routes tell Laravel what to do when a user accesses a particular URL.
web.php
: Contains routes for web pages, handling forms, and returning HTML responses.api.php
: Defines API routes, usually returning JSON data.console.php
: Defines Artisan commands for command-line interactions.channels.php
: Used for broadcasting real-time events and notifications.
8. storage/
– Application Storage
The storage/
folder contains files generated by the application at runtime. These include:
logs/
: Stores application log files.framework/
: Contains cached files, compiled Blade templates, and sessions.app/
: Stores file uploads and user-generated content.
9. tests/
– Application Tests
Laravel encourages testing, and the tests/
folder contains:
- Feature tests: Simulate real user interactions with the application.
- Unit tests: Validate specific application components individually.
Testing ensures that your application functions as expected before deployment.
10. vendor/
– Third-Party Packages
The vendor/
folder contains all your application’s third-party dependencies. Laravel uses Composer to manage PHP packages, and when you run composer install
, all external libraries are placed here.
Since this folder is managed by Composer, you should avoid modifying it manually.
If you haven’t installed Laravel 11 yet, follow our Laravel 11 Installation Guide to get started.“