Mastering Blade Templating in Laravel for Dynamic Websites
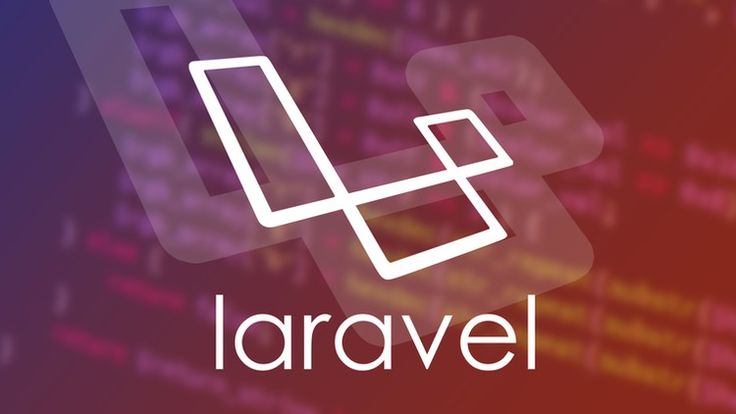
Introduction to Blade Templating in Laravel
Laravel is one of the most popular PHP frameworks for building web applications, and its Blade templating engine is a key component that enhances web development. Blade makes it easy to create dynamic, reusable views for your application, helping developers craft clean, maintainable code.
In this blog post, we will explore what Blade templating is, its features, and how to leverage it for creating SEO-friendly Laravel websites.
What is Blade Templating in Laravel?
Blade is a simple yet powerful templating engine built into Laravel. It allows you to work with HTML and PHP code together in a clean, organized manner. Blade templates have a .blade.php
file extension and are stored in the resources/views
directory of your Laravel application.
Blade is highly flexible, offering features like template inheritance, control structures, and data binding. It’s designed to be simple and intuitive, making it easy for developers to structure and display dynamic content.
Key Features of Blade Templating
-
Template Inheritance: Blade allows you to define a base layout and extend it across multiple views. This is useful for maintaining consistent design and structure across your application. For example, you can create a header, footer, and navigation in one layout file, and then extend it in various views.
Example:
<!– resources/views/layouts/app.blade.php –>
<html>
<head>
<title>@yield(‘title’)</title>
</head>
<body>
<header>@include(‘partials.header’)</header>
<main>@yield(‘content’)</main>
<footer>@include(‘partials.footer’)</footer>
</body>
</html><!– resources/views/welcome.blade.php –>
@extends(‘layouts.app’)@section(‘title’, ‘Welcome Page’)
@section(‘content’)
<h1>Welcome to our website!</h1>
@endsection -
Data Binding: Blade makes it simple to output variables from your controllers directly into views. This ensures that the content is dynamic and can be easily updated based on the data passed to the view.
Example:
<p>Hello, {{ $name }}!</p>
-
Control Structures: Blade provides common PHP control structures like
if
,foreach
, andfor
, but in a cleaner, more readable syntax. These structures make it easy to create dynamic content based on conditions or loops.Example:
@foreach($users as $user)
<p>{{ $user->name }}</p>
@endforeach -
Components & Slots: Blade components allow you to create reusable elements like buttons, cards, or forms, and pass dynamic data to them. Components improve code reusability and maintainability.
Example:
<!– resources/views/components/button.blade.php –>
<button class=”btn btn-primary”>{{ $slot }}</button><!– In another view –>
<x-button>Click Me</x-button> - Blade Directives: Blade comes with built-in directives like
@if
,@foreach
,@include
, and more, which simplify common tasks and improve the readability of your code.
If you’re new to Laravel, you can start with the Laravel Documentation for an in-depth guide on using the framework’s features.