Eloquent ORM in Laravel
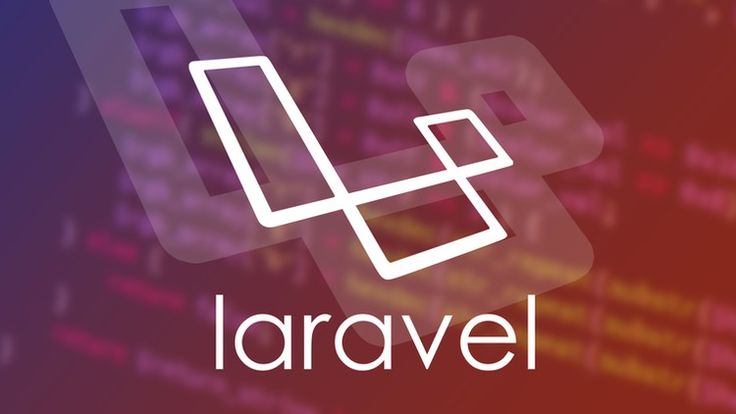
Laravel’s Eloquent ORM (Object-Relational Mapping) is one of the most powerful tools for managing databases in PHP. It provides an expressive, fluent interface to work with databases using Active Record patterns. Whether you’re a beginner or an experienced developer, mastering Eloquent ORM in Laravel can significantly enhance your database management skills.
In this guide, we will explore everything about Laravel Eloquent ORM, from basic queries to advanced relationships and optimizations.
What is Eloquent ORM?
Eloquent is Laravel’s built-in ORM that allows developers to interact with databases using a simple and intuitive syntax. It eliminates the need for raw SQL queries, making database operations cleaner and more readable.
🔹 Key Features of Eloquent ORM:
- Fluent syntax for database queries
- Built-in relationships (One-to-One, One-to-Many, Many-to-Many)
- Soft deletes and timestamps
- Query scopes and eager loading
- Model observers and mutators
👉 For a complete introduction, visit the Laravel Eloquent Documentation.
Step 1: Setting Up Eloquent in Laravel
Eloquent is enabled by default in Laravel. Each database table should have a corresponding model that interacts with it.
To create a model, run:
This command generates a Product
model in app/Models/Product.php
:
The $fillable
property defines mass-assignable fields, preventing mass-assignment vulnerabilities.
Step 2: Basic Database Queries with Eloquent
Retrieving Data
Fetching all records from a table:
Finding a specific record by ID:
Using where()
for filtered queries:
Inserting Data
Creating a new record using Eloquent:
Alternatively, you can use the create()
method:
⚠️ Ensure you define $fillable
in your model to use create()
.
Step 3: Updating and Deleting Records
Updating a Record
To update an existing record:
Alternatively, using update()
:
Deleting a Record
To delete a record using Eloquent:
Or delete using query:
👉 For more details on Eloquent queries, check the Laravel Query Builder Guide.
Step 4: Eloquent Relationships
Eloquent makes managing database relationships easy.
One-to-One Relationship
Example: A user has one profile.
Retrieve user profile:
One-to-Many Relationship
Example: A blog post has many comments.
Retrieve post comments:
Many-to-Many Relationship
Example: A student belongs to many courses.
Retrieve student courses:
👉 Learn more about Eloquent relationships in the Laravel Relationships Guide.
Step 5: Eloquent Advanced Features
Soft Deletes
Enable soft deletes in your model:
Now, deleting a record won’t remove it permanently:
To restore it:
Query Scopes
Define a scope for commonly used queries:
Use it like this:
Mastering Eloquent ORM in Laravel allows you to build efficient, scalable applications with clean and readable database interactions. Whether you’re handling basic queries, relationships, or advanced features like soft deletes and query scopes, Eloquent makes database management easy.
If you need further customization or advanced features, check out our Laravel Services!