How to Build a REST API with Laravel 11
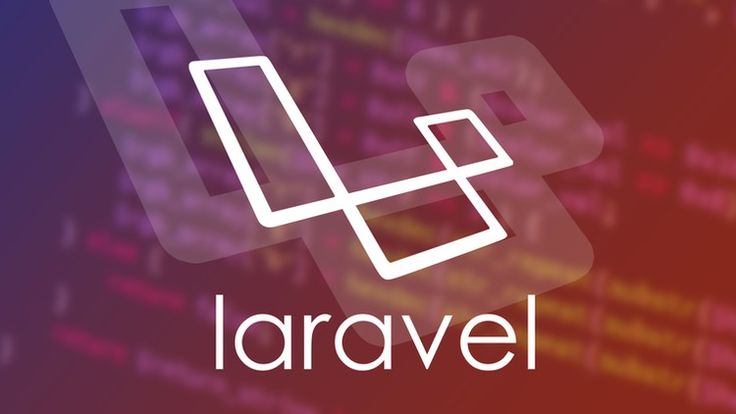
Laravel 11 is one of the most powerful PHP frameworks for building robust and scalable web applications. If you’re looking to create a REST API, Laravel provides built-in support for routing, authentication, and data handling. In this guide, we’ll walk you through the process of setting up a RESTful API in Laravel 11.
Prerequisites
Before you begin, make sure you have the following installed:
- PHP 8.1 or higher
- Composer (Visit Composer to download and install it.)
- Laravel 11
- MySQL or PostgreSQL Database
- Postman (You can download it from Postman for API testing.)
Step 1: Install Laravel 11
To start a new Laravel 11 project, run the following command in your terminal:
composer create-project laravel/laravel laravel11-api
Navigate into your project directory:
cd laravel11-api
Step 2: Set Up Database Configuration
Open the .env
file and configure your database settings:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_api
DB_USERNAME=root
DB_PASSWORD=
Then, run the migration command to create default tables:
php artisan migrate
For more details, visit the Laravel Migration Guide.
Step 3: Create a Model and Migration
Generate a new model with a migration file:
php artisan make:model Post -m
Modify the database/migrations/xxxx_xx_xx_create_posts_table.php
file to define the schema:
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
Run the migration to create the posts
table:
php artisan migrate
Step 4: Create a RESTful Controller
Generate a new controller for handling API requests:
php artisan make:controller Api/PostController --api
Modify app/Http/Controllers/Api/PostController.php
:
use App\Models\Post;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
class PostController extends Controller
{
public function index()
{
return response()->json(Post::all(), 200);
}
public function store(Request $request)
{
$request->validate([
'title' => 'required',
'content' => 'required',
]);
$post = Post::create($request->all());
return response()->json($post, 201);
}
public function show(Post $post)
{
return response()->json($post, 200);
}
public function update(Request $request, Post $post)
{
$post->update($request->all());
return response()->json($post, 200);
}
public function destroy(Post $post)
{
$post->delete();
return response()->json(null, 204);
}
}
Step 5: Define API Routes
Edit routes/api.php
to include the API routes:
use App\Http\Controllers\Api\PostController;
Route::apiResource('posts', PostController::class);
For more details on API routing, check out the Laravel Routing Documentation.
Step 6: Test Your API
Start your Laravel server:
php artisan serve
Use Postman or CURL to test your API endpoints:
GET http://127.0.0.1:8000/api/posts
POST http://127.0.0.1:8000/api/posts
Content-Type: application/json
{
"title": "New Post",
"content": "This is the content of the post."
}
Step 7: Implement Authentication (Optional)
To secure your API, you can use Laravel Sanctum:
composer require laravel/sanctum
Publish and run the migration:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
php artisan migrate
Register Sanctum’s middleware in app/Http/Kernel.php
:
'api' => [
\Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
Modify app/Models/User.php
to use the HasApiTokens trait:
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable;
}
For more information on authentication, visit the Laravel Sanctum Guide.
Congratulations! You have successfully built a REST API with Laravel 11. You can now extend this API by adding authentication, validation, and advanced features.
If you need further customization or advanced features, check out our Laravel Services!