Laravel MVC Architecture
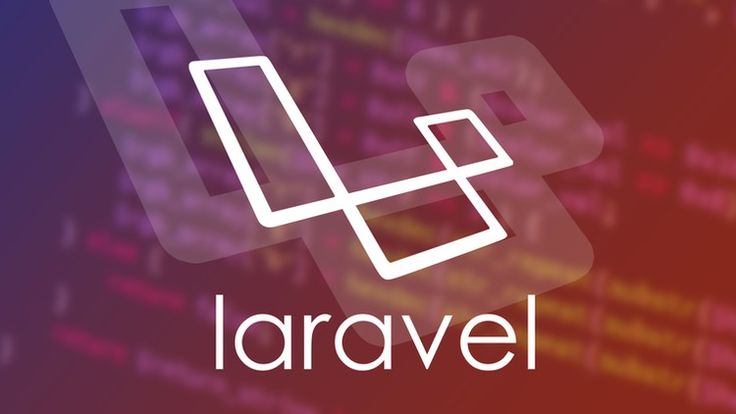
Introduction: What is Laravel MVC Architecture?
Laravel MVC (Model-View-Controller) is a popular architecture pattern used to structure web applications. It divides the application into three core components: Model, View, and Controller, ensuring separation of concerns. This structure makes it easier to manage, maintain, and scale your application in Laravel. Whether you’re a beginner or an experienced developer, understanding Laravel’s MVC architecture will enhance your development workflow.
What is MVC Architecture?
MVC stands for Model-View-Controller, a design pattern that divides an application into three interconnected components:
-
Model: Represents the application’s data structure. It interacts with the database and handles data logic, such as querying and updating records.
-
View: The user interface. This is what users interact with – HTML, CSS, JavaScript – everything that’s displayed on the frontend of the application.
-
Controller: Acts as an intermediary between the Model and the View. It receives user input, processes it (with the help of the Model), and returns the output to the View.
How Laravel Implements MVC
In Laravel, the implementation of MVC is straightforward and intuitive. Let’s break it down further:
1. Model in Laravel
Models in Laravel are typically represented as Eloquent ORM classes. Eloquent provides a simple and elegant syntax for interacting with your database. Each model corresponds to a table in the database, and it defines relationships between tables.
For example, you can define a Post
model for a blog, which can retrieve, update, and manipulate data in the posts
table.
class Post extends Model {
protected $table = ‘posts’;
}
This model could then be used to fetch or insert data, simplifying complex database interactions. For more information on working with models, visit Laravel’s official Eloquent documentation.
2. View in Laravel
Views in Laravel are typically written in Blade, Laravel’s templating engine. Blade allows you to combine PHP code with HTML for a dynamic frontend. Views display the data passed from the controller to the user.
For instance, when you pass data from a controller to a view:
The post.show
Blade view might look something like this:
This view will render the post’s title and content dynamically.
3. Controller in Laravel
Controllers in Laravel handle user input and return the corresponding response. The controller fetches the relevant model data and returns it to the view.
Here’s a simple example of a PostController that handles displaying a single post:
The controller communicates with the Post model to retrieve the data, and then passes that data to the view for display.
Benefits of MVC in Laravel
The MVC architecture in Laravel provides several benefits for developers:
-
Separation of Concerns: Each component (Model, View, Controller) has a clear responsibility, making it easier to manage and debug.
-
Reusability: Since models are separate from views, they can be reused in multiple places within the application.
-
Maintainability: With clear separation, it’s easier to maintain and scale your application as it grows.
-
Cleaner Code: The MVC pattern leads to cleaner, more organized code, making it easier to understand and develop.
Key Laravel Features for MVC
Laravel comes with several features that work seamlessly with the MVC architecture:
- Routing: Laravel’s powerful routing system allows you to easily map URL requests to specific controllers.
- Middleware: You can add layers of functionality, such as authentication, before requests reach your controllers.
- Blade Templating: Laravel’s Blade templating engine makes it simple to render views with dynamic content.
For further reading on Laravel’s core features, check out the official Laravel documentation.
Best Practices for Working with MVC in Laravel
- Keep Controllers Thin: Don’t overload your controllers with logic. Instead, use services or repositories for business logic.
- Eloquent Relationships: Use Eloquent relationships to simplify working with related models (e.g., one-to-many, many-to-many).
- Use Dependency Injection: Laravel makes it easy to inject dependencies into controllers, which improves testability and decoupling.
Understanding the Laravel MVC architecture is key to building efficient, maintainable web applications. By separating concerns into Models, Views, and Controllers, Laravel provides a structured way to organize your application code. Whether you’re a beginner or an experienced developer, understanding MVC will help you create cleaner, more scalable applications.
If you’re new to Laravel, you can start with the Laravel Documentation for an in-depth guide on using the framework’s features.
To dive deeper, explore the Laravel 11 Guide for the latest updates and best practices.