Laravel Routing Guide: Learn to Create Routes in Laravel
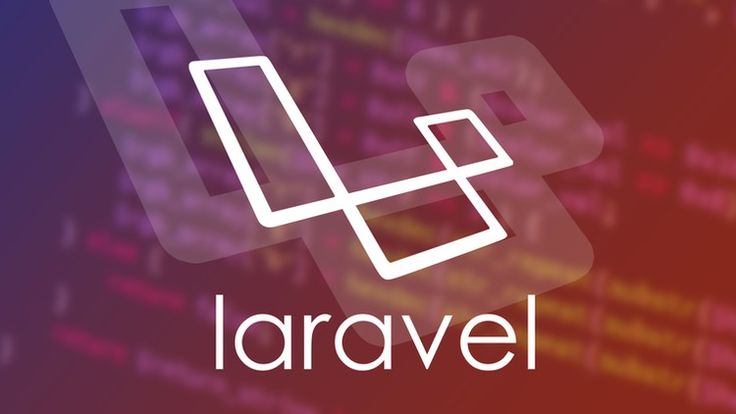
Routing is a crucial part of web development, and in Laravel, it’s both powerful and flexible. Whether you’re a beginner or looking to brush up on Laravel routing, this guide will help you learn how to define, manage, and customize routes for your Laravel applications.
What is Routing in Laravel?
In Laravel, routes are the foundation of a web application’s URL structure. They act as the entry point for handling HTTP requests and directing them to the appropriate controller or action. Laravel’s routing system is highly intuitive and flexible, allowing developers to quickly set up routes for various HTTP methods like GET
, POST
, PUT
, and DELETE
.
How to Define Routes in Laravel
Defining routes in Laravel is simple and done within the routes/web.php
file. Here’s how you can define a basic route:
Route::get(‘/welcome’, function () {
return view(‘welcome’);
});
In this example, when a user visits the /welcome
URL in their browser, the route returns the welcome
view.
Laravel Route Parameters
Laravel routes can accept parameters, allowing you to capture values from the URL. For example:
Route::get(‘/user/{id}’, function ($id) {
return ‘User ID is ‘ . $id;
});
In this case, the {id}
parameter will capture any value passed in the URL, and you can access it inside your route callback.
Route Groups in Laravel
To organize routes and apply middleware or shared attributes, Laravel allows you to group routes. For instance, you can group all routes that need authentication:
Route::middleware(‘auth’)->group(function () {
Route::get(‘/dashboard’, function () {
return view(‘dashboard’);
});
});
With this, any route within the group requires the user to be authenticated.
Named Routes in Laravel
Named routes allow you to refer to routes by their names instead of hardcoding URLs. This can be particularly useful when generating URLs within views or redirecting users.
Route::get(‘/profile’, function () {
// Profile page
})->name(‘profile’);
$url = route(‘profile’);
Resource Controllers in Laravel
Laravel makes it easy to work with resourceful routes. A resource controller automatically defines routes for actions like index
, store
, show
, edit
, and update
. You can generate these routes by using the Route::resource
method.
Route::resource(‘posts’, PostController::class);
This will automatically generate routes for all the basic actions needed to manage posts
.
Route Middleware in Laravel
Route middleware allows you to run code before or after a route request is handled. For example, you can use middleware to authenticate users or log requests.
Route::get(‘/profile’, function () {
// Profile page
})->middleware(‘auth’);
The auth
middleware ensures that only authenticated users can access this route.
Dynamic Routing in Laravel
Sometimes, you need dynamic routing where the URL structure changes based on specific conditions. You can create such routes by utilizing regular expressions or route parameters.
Route::get(‘/category/{slug}’, function ($slug) {
// Display category by slug
});
In this example, {slug}
is dynamic, and it can be any value that represents a category’s unique slug.
Understanding routing in Laravel is essential to building a structured and scalable web application. With the flexibility of route parameters, middleware, groups, and named routes, you can easily manage your app’s URL structure. By following the best practices discussed in this guide, you’ll be able to create efficient and maintainable routes for your Laravel projects.
If you want to see an example of how Laravel routing works in a CRUD system, check out my Laravel CRUD System GitHub repository where I demonstrate the use of routes, controllers, and views to build a complete application.
Learn about Laravel MVC, visit Laravel MVC guide
- Official Laravel Routing Documentation: Laravel Routing Docs
- Tutorial on Laravel Controllers: How to Use Laravel Controllers